删除链表的倒数第N个结点
力扣题号19
题目描述:
1
| 给你一个链表,删除链表的倒数第 n 个结点,并且返回链表的头结点。
|
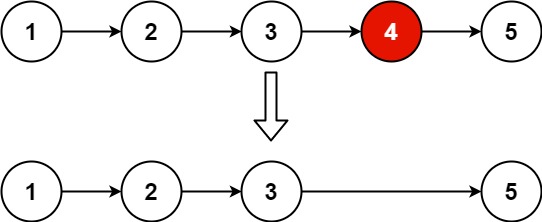
1 2 3 4 5 6 7 8 9 10
| 输入:head = [1,2,3,4,5], n = 2 输出:[1,2,3,5] 示例 2:
输入:head = [1], n = 1 输出:[] 示例 3:
输入:head = [1,2], n = 1 输出:[1]
|
题目不难,考察对链表结构的掌握,因为链表没有记录长度,所以需要我们去计算这个索引的位置,最好的方法就是快慢指针
快慢指针
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { ListNode header = new ListNode(-1); header.next = head; ListNode fast = header; ListNode slow = header; while (n-- >0 && fast.next != null){ fast = fast.next; } while (fast.next != null){ fast = fast.next; slow = slow.next; } assert slow.next != null; slow.next = slow.next.next; return header.next; } }
|
node结构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class ListNode { int val; ListNode next;
ListNode() { }
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; } }
|